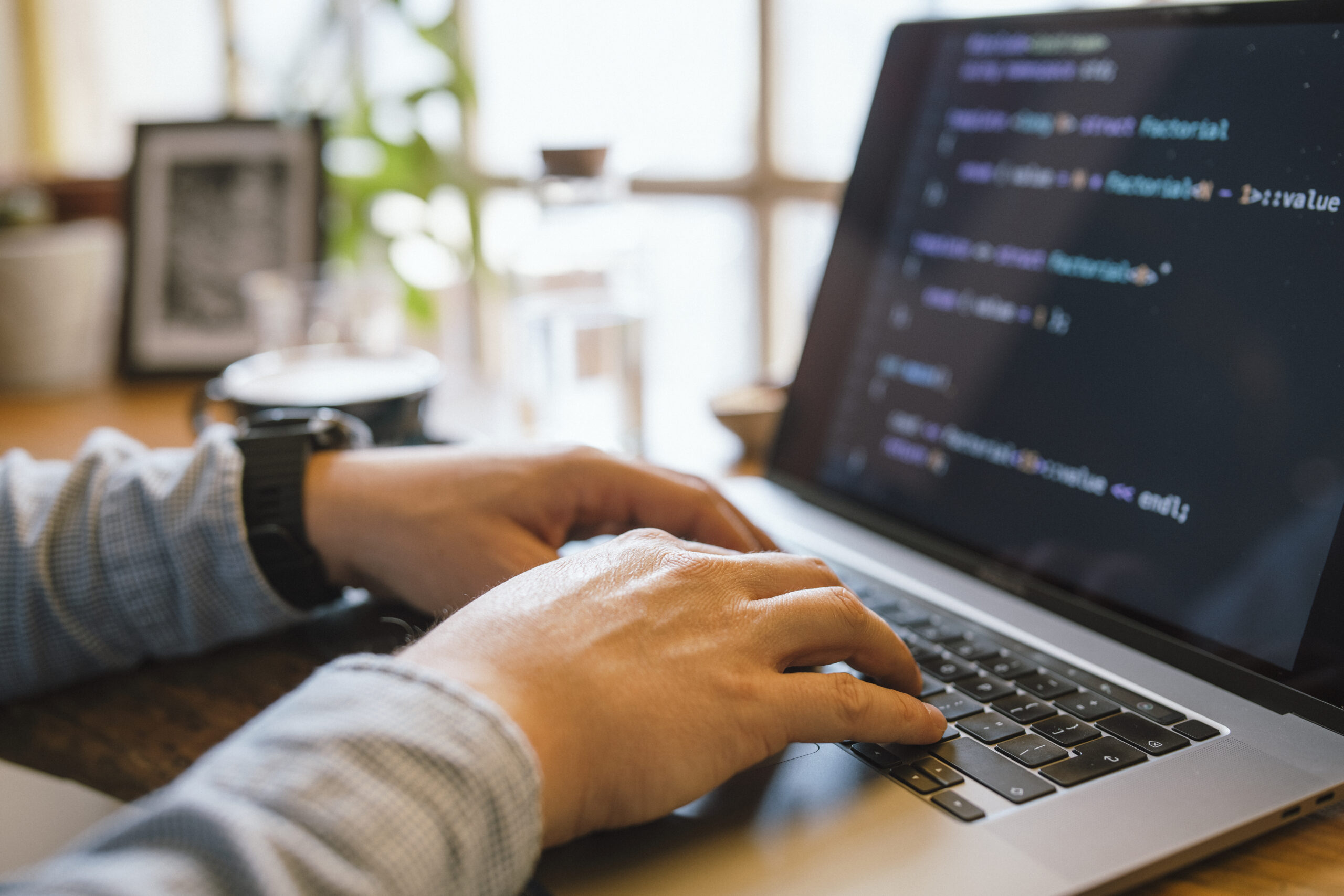
Debugging is One of the more critical — but typically forgotten — skills inside a developer’s toolkit. It isn't pretty much correcting damaged code; it’s about being familiar with how and why things go Incorrect, and Studying to Feel methodically to resolve difficulties effectively. No matter whether you're a novice or even a seasoned developer, sharpening your debugging expertise can conserve hours of aggravation and significantly enhance your productivity. Here are several procedures that will help builders degree up their debugging sport by me, Gustavo Woltmann.
Grasp Your Resources
One of the fastest approaches developers can elevate their debugging abilities is by mastering the tools they use everyday. When composing code is 1 part of enhancement, figuring out the best way to interact with it correctly through execution is equally important. Modern-day advancement environments come Outfitted with potent debugging abilities — but a lot of developers only scratch the area of what these equipment can perform.
Just take, for instance, an Built-in Advancement Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These tools help you set breakpoints, inspect the worth of variables at runtime, step by code line by line, and also modify code over the fly. When employed correctly, they Allow you to notice specifically how your code behaves all through execution, which can be a must have for tracking down elusive bugs.
Browser developer equipment, such as Chrome DevTools, are indispensable for front-end developers. They assist you to inspect the DOM, check community requests, see authentic-time efficiency metrics, and debug JavaScript during the browser. Mastering the console, sources, and community tabs can flip discouraging UI problems into workable tasks.
For backend or technique-level developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB present deep Manage about running processes and memory management. Finding out these applications may have a steeper Understanding curve but pays off when debugging performance concerns, memory leaks, or segmentation faults.
Outside of your IDE or debugger, become cozy with Model Regulate units like Git to know code historical past, uncover the precise minute bugs ended up released, and isolate problematic variations.
Ultimately, mastering your resources implies heading over and above default options and shortcuts — it’s about establishing an intimate understanding of your growth natural environment making sure that when difficulties crop up, you’re not missing at the hours of darkness. The greater you are aware of your applications, the greater time you may shell out fixing the actual difficulty instead of fumbling via the process.
Reproduce the Problem
One of the more significant — and infrequently neglected — measures in successful debugging is reproducing the issue. Before leaping in the code or generating guesses, developers need to produce a regular surroundings or scenario where by the bug reliably seems. With no reproducibility, fixing a bug results in being a match of probability, typically leading to squandered time and fragile code adjustments.
The first step in reproducing a challenge is gathering just as much context as you can. Inquire thoughts like: What actions led to The problem? Which atmosphere was it in — enhancement, staging, or creation? Are there any logs, screenshots, or error messages? The more element you might have, the simpler it will become to isolate the exact ailments below which the bug takes place.
As soon as you’ve gathered ample information, try and recreate the problem in your neighborhood environment. This might necessarily mean inputting the identical details, simulating equivalent person interactions, or mimicking method states. If The problem seems intermittently, think about producing automatic tests that replicate the edge conditions or state transitions included. These checks not only support expose the condition but additionally protect against regressions Down the road.
At times, The difficulty might be setting-precise — it might occur only on specified functioning systems, browsers, or below distinct configurations. Working with tools like virtual machines, containerization (e.g., Docker), or cross-browser screening platforms might be instrumental in replicating these bugs.
Reproducing the problem isn’t just a stage — it’s a attitude. It calls for patience, observation, along with a methodical strategy. But as soon as you can continually recreate the bug, you might be now midway to correcting it. Which has a reproducible state of affairs, You should use your debugging resources much more efficiently, check prospective fixes securely, and talk much more clearly together with your group or customers. It turns an abstract criticism right into a concrete problem — and that’s in which developers thrive.
Browse and Have an understanding of the Mistake Messages
Error messages are frequently the most precious clues a developer has when some thing goes Incorrect. Rather than looking at them as discouraging interruptions, builders must discover to treat mistake messages as immediate communications through the program. They frequently tell you what precisely took place, in which it happened, and in some cases even why it transpired — if you understand how to interpret them.
Commence by studying the information meticulously and in full. Quite a few developers, especially when underneath time strain, glance at the primary line and right away start generating assumptions. But deeper from the error stack or logs may lie the genuine root trigger. Don’t just duplicate and paste error messages into search engines — examine and realize them to start with.
Crack the error down into areas. Is it a syntax error, a runtime exception, or a logic error? Will it stage to a certain file and line number? What module or operate brought on it? These queries can guideline your investigation and level you towards the responsible code.
It’s also beneficial to be familiar with the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically abide by predictable patterns, and Finding out to acknowledge these can significantly accelerate your debugging system.
Some problems are imprecise or generic, As well as in These situations, it’s very important to examine the context during which the mistake happened. Look at associated log entries, input values, and up to date improvements in the codebase.
Don’t neglect compiler or linter warnings both. These generally precede larger sized problems and provide hints about prospective bugs.
In the long run, mistake messages are usually not your enemies—they’re your guides. Studying to interpret them appropriately turns chaos into clarity, supporting you pinpoint difficulties a lot quicker, reduce debugging time, and become a more efficient and assured developer.
Use Logging Properly
Logging is Just about the most highly effective instruments inside of a developer’s debugging toolkit. When made use of effectively, it offers serious-time insights into how an software behaves, encouraging you have an understanding of what’s going on underneath the hood without having to pause execution or action from the code line by line.
A fantastic logging approach commences with being aware of what to log and at what degree. Frequent logging ranges consist of DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for specific diagnostic facts for the duration of advancement, Information for common events (like thriving start out-ups), WARN for prospective issues that don’t crack the applying, ERROR for actual complications, and Deadly once the system can’t continue on.
Keep away from flooding your logs with extreme or irrelevant data. Far too much logging can obscure critical messages and slow down your procedure. Target crucial events, condition modifications, enter/output values, and demanding decision details within your code.
Structure your log messages Obviously and consistently. Include things like context, like timestamps, ask for IDs, and function names, so it’s much easier to trace problems in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
All through debugging, logs Allow you to keep track of how variables evolve, what problems are met, and what branches of logic are executed—all with no halting This system. They’re Specifically important in manufacturing environments where by stepping via code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about stability and clarity. Which has a nicely-considered-out logging approach, you'll be able to lessen the time it takes to spot troubles, attain deeper visibility into your programs, and Enhance the In general maintainability and reliability of the code.
Assume Similar to a Detective
Debugging is not just a specialized process—it is a method of investigation. To successfully recognize and resolve bugs, builders ought to approach the process like a detective fixing a thriller. This frame of mind helps break down sophisticated troubles into workable sections and abide by clues logically to uncover the foundation cause.
Begin by gathering evidence. Look at the signs of the trouble: error messages, incorrect output, or functionality troubles. The same as a detective surveys against the law scene, accumulate just as much suitable facts as you may devoid of leaping to conclusions. Use logs, take a look at situations, and consumer studies to piece collectively a clear picture of what’s going on.
Next, form hypotheses. Talk to you: What can be resulting in this habits? Have any alterations not too long ago been produced to the codebase? Has this difficulty happened ahead of below comparable circumstances? The intention will be to slim down choices and detect probable culprits.
Then, examination your theories systematically. Attempt to recreate the problem in a very controlled atmosphere. For those who suspect a certain function or ingredient, isolate it and confirm if The difficulty persists. Just like a detective conducting interviews, inquire your code thoughts and Allow the results guide you closer to the reality.
Spend shut focus to small aspects. Bugs typically hide while in the least predicted locations—similar to a missing semicolon, an off-by-one particular error, or possibly a race situation. Be extensive and patient, resisting the urge to patch The problem without entirely understanding it. Momentary fixes may conceal the actual issue, just for it to resurface afterwards.
Finally, retain notes on what you experimented with and acquired. Just as detectives log their investigations, documenting your debugging approach can save time for long run problems and support Many others realize your reasoning.
By imagining similar to a detective, developers can sharpen their analytical expertise, tactic problems methodically, and grow to be simpler at uncovering concealed problems in sophisticated devices.
Write Tests
Composing checks is one of the best solutions to enhance your debugging expertise and General growth performance. Tests not only aid catch bugs early but in addition function a security Internet that provides you self esteem when earning changes for your codebase. A nicely-tested application is easier to debug because it enables you to pinpoint specifically in which and when a difficulty happens.
Start with unit tests, which focus on individual functions or modules. These compact, isolated checks can immediately expose no matter if a Developers blog certain bit of logic is Functioning as anticipated. Whenever a check fails, you immediately know where to glimpse, appreciably cutting down enough time invested debugging. Unit checks are In particular valuable for catching regression bugs—concerns that reappear following Beforehand staying fastened.
Up coming, integrate integration checks and conclude-to-stop tests into your workflow. These enable be certain that numerous parts of your software function alongside one another efficiently. They’re specifically useful for catching bugs that come about in sophisticated systems with many elements or products and services interacting. If anything breaks, your tests can inform you which A part of the pipeline unsuccessful and below what disorders.
Composing checks also forces you to Imagine critically about your code. To check a characteristic properly, you require to know its inputs, predicted outputs, and edge instances. This standard of comprehending Obviously prospects to higher code composition and fewer bugs.
When debugging an issue, creating a failing take a look at that reproduces the bug may be a strong starting point. Once the examination fails consistently, you'll be able to deal with fixing the bug and observe your take a look at pass when the issue is solved. This solution ensures that the identical bug doesn’t return Sooner or later.
To put it briefly, creating exams turns debugging from the disheartening guessing game into a structured and predictable approach—serving to you capture more bugs, more quickly and a lot more reliably.
Choose Breaks
When debugging a tough issue, it’s straightforward to become immersed in the challenge—observing your monitor for several hours, seeking solution following Remedy. But Among the most underrated debugging applications is solely stepping absent. Having breaks allows you reset your intellect, cut down frustration, and often see The difficulty from the new standpoint.
If you're far too near the code for way too very long, cognitive tiredness sets in. You could possibly start off overlooking clear problems or misreading code that you just wrote just hrs earlier. Within this state, your Mind will become a lot less productive at difficulty-solving. A brief wander, a espresso break, or perhaps switching to a different endeavor for ten–15 minutes can refresh your concentrate. Many builders report obtaining the basis of a problem when they've taken time and energy to disconnect, letting their subconscious work during the qualifications.
Breaks also aid stop burnout, Primarily through more time debugging sessions. Sitting down in front of a monitor, mentally trapped, is not merely unproductive but also draining. Stepping absent permits you to return with renewed energy and also a clearer attitude. You might quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you before.
When you’re stuck, a fantastic rule of thumb should be to set a timer—debug actively for forty five–60 minutes, then take a five–10 moment break. Use that time to maneuver close to, extend, or do one thing unrelated to code. It may well really feel counterintuitive, In particular below limited deadlines, however it in fact leads to more rapidly and more practical debugging Over time.
Briefly, taking breaks is just not an indication of weakness—it’s a wise tactic. It provides your Mind space to breathe, improves your point of view, and allows you avoid the tunnel vision That always blocks your development. Debugging is really a mental puzzle, and relaxation is an element of solving it.
Find out From Just about every Bug
Every bug you experience is much more than simply a temporary setback—It is a chance to improve to be a developer. Whether or not it’s a syntax error, a logic flaw, or possibly a deep architectural difficulty, every one can teach you some thing useful when you go to the trouble to replicate and analyze what went Incorrect.
Commence by asking by yourself some vital questions once the bug is resolved: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with much better methods like unit testing, code critiques, or logging? The answers frequently reveal blind places in the workflow or being familiar with and help you build much better coding patterns going ahead.
Documenting bugs can be a fantastic routine. Preserve a developer journal or sustain a log where you note down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see styles—recurring challenges or prevalent problems—which you can proactively steer clear of.
In team environments, sharing Anything you've figured out from a bug together with your friends is often Specifically potent. Whether it’s via a Slack concept, a brief publish-up, or a quick knowledge-sharing session, serving to Other folks avoid the exact situation boosts team performance and cultivates a more powerful learning lifestyle.
Much more importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as necessary elements of your enhancement journey. All things considered, some of the finest developers will not be those who compose fantastic code, but individuals who consistently find out from their issues.
Ultimately, Each individual bug you resolve provides a new layer to the talent set. So following time you squash a bug, have a moment to mirror—you’ll appear absent a smarter, much more able developer thanks to it.
Conclusion
Strengthening your debugging competencies requires time, exercise, and tolerance — however the payoff is big. It would make you a more effective, self-confident, and able developer. Another time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to be much better at Whatever you do.