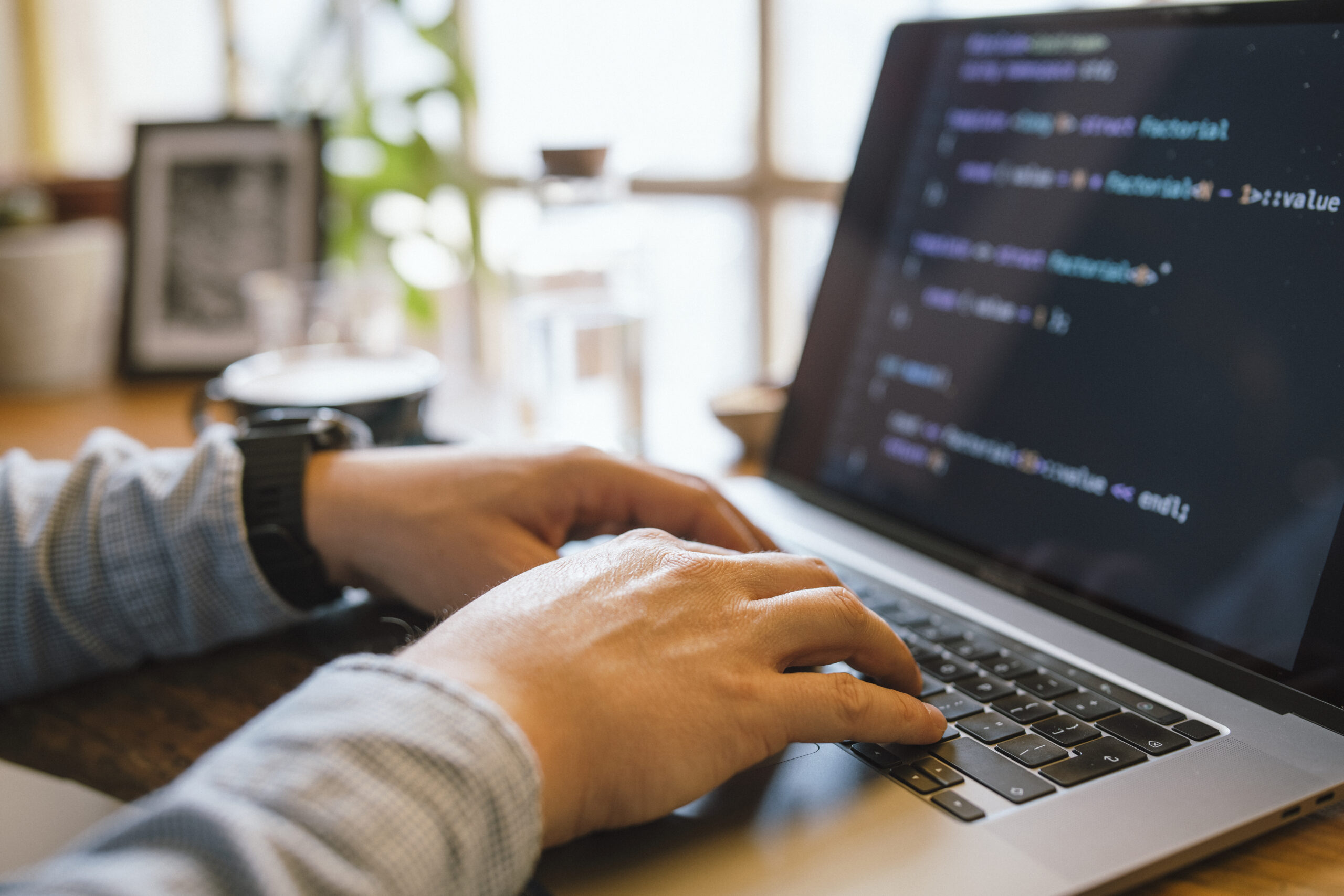
Debugging is Probably the most important — still normally ignored — expertise in the developer’s toolkit. It's actually not pretty much correcting damaged code; it’s about understanding how and why issues go Incorrect, and Understanding to Feel methodically to resolve challenges competently. Whether you are a starter or simply a seasoned developer, sharpening your debugging competencies can help you save several hours of irritation and radically help your efficiency. Here's various tactics to help you developers degree up their debugging game by me, Gustavo Woltmann.
Learn Your Applications
Among the list of quickest methods developers can elevate their debugging competencies is by mastering the instruments they use each day. When composing code is just one Section of advancement, understanding how to connect with it properly throughout execution is Similarly significant. Modern day improvement environments occur Outfitted with potent debugging abilities — but a lot of developers only scratch the area of what these equipment can do.
Choose, by way of example, an Integrated Progress Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments allow you to established breakpoints, inspect the value of variables at runtime, move by code line by line, and in many cases modify code about the fly. When applied effectively, they Enable you to notice exactly how your code behaves during execution, which happens to be priceless for monitoring down elusive bugs.
Browser developer instruments, including Chrome DevTools, are indispensable for front-close developers. They assist you to inspect the DOM, keep an eye on network requests, perspective true-time functionality metrics, and debug JavaScript within the browser. Mastering the console, resources, and network tabs can switch irritating UI difficulties into workable duties.
For backend or process-level developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB offer you deep control around jogging procedures and memory management. Discovering these equipment can have a steeper learning curve but pays off when debugging functionality difficulties, memory leaks, or segmentation faults.
Beyond your IDE or debugger, grow to be at ease with Variation control techniques like Git to be aware of code record, find the exact second bugs have been launched, and isolate problematic improvements.
Finally, mastering your tools indicates going beyond default settings and shortcuts — it’s about creating an intimate understanding of your advancement environment to ensure that when problems arise, you’re not misplaced at midnight. The better you understand your equipment, the greater time it is possible to shell out fixing the actual difficulty in lieu of fumbling by the method.
Reproduce the situation
One of the more significant — and infrequently missed — techniques in productive debugging is reproducing the trouble. Just before jumping in to the code or making guesses, builders have to have to produce a dependable ecosystem or state of affairs wherever the bug reliably seems. With no reproducibility, repairing a bug turns into a recreation of opportunity, usually resulting in wasted time and fragile code modifications.
The first step in reproducing a dilemma is collecting as much context as feasible. Check with queries like: What steps triggered The problem? Which ecosystem was it in — progress, staging, or creation? Are there any logs, screenshots, or mistake messages? The more detail you may have, the less difficult it gets to be to isolate the precise circumstances less than which the bug happens.
As you’ve collected more than enough data, attempt to recreate the condition in your local natural environment. This could imply inputting a similar info, simulating identical user interactions, or mimicking process states. If the issue appears intermittently, take into consideration creating automated assessments that replicate the edge circumstances or point out transitions involved. These assessments not only assistance expose the trouble and also stop regressions Later on.
In some cases, the issue could possibly be ecosystem-distinct — it'd take place only on selected functioning methods, browsers, or underneath individual configurations. Utilizing instruments like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this sort of bugs.
Reproducing the condition isn’t just a phase — it’s a mentality. It demands persistence, observation, plus a methodical solution. But once you can constantly recreate the bug, you happen to be now halfway to repairing it. That has a reproducible state of affairs, you can use your debugging resources a lot more properly, take a look at probable fixes safely and securely, and converse additional Evidently with all your workforce or buyers. It turns an summary criticism right into a concrete problem — and that’s in which developers prosper.
Browse and Have an understanding of the Mistake Messages
Mistake messages are sometimes the most useful clues a developer has when something goes wrong. Rather than looking at them as discouraging interruptions, builders should really discover to treat error messages as immediate communications from the method. They frequently tell you just what occurred, where it happened, and in some cases even why it took place — if you understand how to interpret them.
Start off by looking at the concept very carefully and in comprehensive. Quite a few builders, particularly when below time tension, look at the very first line and immediately get started generating assumptions. But deeper from the error stack or logs may lie the genuine root trigger. Don’t just duplicate and paste error messages into search engines like yahoo — go through and comprehend them to start with.
Split the mistake down into areas. Is it a syntax error, a runtime exception, or a logic error? Will it level to a selected file and line amount? What module or function induced it? These questions can manual your investigation and place you toward the dependable code.
It’s also helpful to be aware of the terminology from the programming language or framework you’re working with. Error messages in languages like Python, JavaScript, or Java usually abide by predictable patterns, and Finding out to recognize these can considerably quicken your debugging approach.
Some errors are obscure or generic, As well as in Those people instances, it’s important to look at the context by which the error occurred. Check out similar log entries, input values, and recent alterations during the codebase.
Don’t neglect compiler or linter warnings both. These generally precede larger sized challenges and provide hints about prospective bugs.
In the long run, mistake messages are usually not your enemies—they’re your guides. Studying to interpret them appropriately turns chaos into clarity, supporting you pinpoint difficulties a lot quicker, decrease debugging time, and become a a lot more productive and self-confident developer.
Use Logging Correctly
Logging is One of the more powerful tools inside a developer’s debugging toolkit. When utilized efficiently, it provides actual-time insights into how an application behaves, aiding you realize what’s taking place beneath the hood with no need to pause execution or stage with the code line by line.
A great logging technique starts with understanding what to log and at what level. Prevalent logging degrees include things like DEBUG, Details, WARN, ERROR, and FATAL. Use DEBUG for detailed diagnostic information all through enhancement, Details for standard functions (like profitable commence-ups), WARN for potential challenges that don’t split the appliance, ERROR for precise challenges, and Deadly when the procedure can’t continue on.
Keep away from flooding your logs with excessive or irrelevant details. An excessive amount logging can obscure critical messages and slow down your procedure. Target crucial events, point out adjustments, enter/output values, and demanding decision details within your code.
Structure your log messages Plainly and consistently. Include things like context, including timestamps, request IDs, and performance names, so it’s easier to trace challenges in distributed systems or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even easier to parse and filter logs programmatically.
For the duration of debugging, logs let you observe how variables evolve, what circumstances are fulfilled, and what branches of logic are executed—all with out halting This system. They’re Particularly precious in manufacturing environments wherever stepping via code isn’t doable.
Furthermore, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with checking dashboards.
Finally, smart logging is about equilibrium and clarity. Having a properly-assumed-out logging strategy, you could reduce the time it will require to spot troubles, attain deeper visibility into your apps, and Increase the All round maintainability and trustworthiness of your code.
Consider Similar to a Detective
Debugging is not just a specialized process—it is a form of investigation. To properly determine and deal with bugs, developers ought to method the method similar to a detective solving a mystery. This frame of mind allows break down complicated troubles into workable pieces and follow clues logically to uncover the root result in.
Start off by collecting proof. Look at the signs and symptoms of the situation: mistake messages, incorrect output, or overall performance troubles. The same as a detective surveys a crime scene, collect as much related details as you may devoid of leaping to conclusions. Use logs, exam cases, and user reviews to piece collectively a transparent photo of what’s occurring.
Upcoming, variety hypotheses. Talk to yourself: What could possibly be resulting in this actions? Have any changes not too long ago been created for the codebase? Has this concern transpired just before beneath very similar situation? The purpose is usually to narrow down options and establish likely culprits.
Then, examination your theories systematically. Make an effort to recreate the issue inside of a managed surroundings. In the event you suspect a selected purpose or element, isolate it and validate if The problem persists. Just like a detective conducting interviews, ask your code thoughts and Permit the outcome lead you nearer to the truth.
Pay back near interest to small facts. Bugs usually hide from the least envisioned areas—similar to a missing semicolon, an off-by-one mistake, or perhaps a race affliction. Be comprehensive and affected individual, resisting the urge to patch The problem without entirely comprehending it. Non permanent fixes may cover the actual difficulty, just for it to resurface later.
And lastly, maintain notes on Anything you attempted and figured out. Just as detectives log their investigations, documenting your debugging approach can help you save time for potential challenges and assist Some others comprehend your reasoning.
By contemplating similar to a detective, developers can sharpen their analytical capabilities, solution issues methodically, and become simpler at uncovering concealed challenges in complicated techniques.
Generate Tests
Creating assessments is among the simplest methods to increase your debugging competencies and overall improvement effectiveness. Assessments not simply assistance capture Developers blog bugs early but also serve as a safety Web that offers you confidence when creating alterations to the codebase. A very well-analyzed software is much easier to debug mainly because it helps you to pinpoint specifically the place and when a challenge happens.
Begin with device assessments, which target particular person capabilities or modules. These smaller, isolated checks can promptly expose irrespective of whether a selected bit of logic is Performing as predicted. Every time a take a look at fails, you quickly know the place to seem, substantially lowering time spent debugging. Device assessments are In particular valuable for catching regression bugs—troubles that reappear right after previously being preset.
Future, combine integration exams and end-to-end assessments into your workflow. These support make certain that numerous aspects of your software operate with each other effortlessly. They’re specially beneficial for catching bugs that happen in elaborate devices with several components or expert services interacting. If one thing breaks, your tests can inform you which A part of the pipeline unsuccessful and beneath what circumstances.
Crafting assessments also forces you to Imagine critically regarding your code. To test a aspect appropriately, you'll need to understand its inputs, predicted outputs, and edge instances. This standard of comprehending The natural way prospects to raised code structure and less bugs.
When debugging an issue, composing a failing exam that reproduces the bug could be a robust first step. After the exam fails constantly, you could concentrate on repairing the bug and check out your check move when The difficulty is resolved. This technique makes certain that exactly the same bug doesn’t return Sooner or later.
To put it briefly, creating exams turns debugging from the frustrating guessing sport right into a structured and predictable course of action—helping you catch far more bugs, a lot quicker and much more reliably.
Just take Breaks
When debugging a tough difficulty, it’s simple to become immersed in the challenge—observing your monitor for several hours, attempting Remedy soon after Option. But one of the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, lessen disappointment, and sometimes see The problem from the new standpoint.
If you're much too near the code for too long, cognitive fatigue sets in. You may commence overlooking apparent mistakes or misreading code that you simply wrote just hours earlier. Within this state, your brain becomes much less effective at issue-solving. A brief stroll, a coffee break, or perhaps switching to a different endeavor for ten–quarter-hour can refresh your emphasis. Several developers report finding the foundation of a dilemma once they've taken time for you to disconnect, letting their subconscious work during the qualifications.
Breaks also support avoid burnout, Particularly during for a longer period debugging periods. Sitting before a display screen, mentally stuck, is not only unproductive but will also draining. Stepping away enables you to return with renewed energy and also a clearer attitude. You may instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you ahead of.
In the event you’re trapped, a great general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–10 minute crack. Use that time to maneuver close to, extend, or do one thing unrelated to code. It may well come to feel counterintuitive, especially underneath tight deadlines, but it surely really causes more quickly and more practical debugging Over time.
To put it briefly, taking breaks will not be an indication of weak point—it’s a wise strategy. It provides your Mind space to breathe, enhances your standpoint, and aids you steer clear of the tunnel vision That usually blocks your development. Debugging is usually a mental puzzle, and rest is an element of resolving it.
Discover From Just about every Bug
Every bug you come across is a lot more than simply a temporary setback—It really is a possibility to grow as being a developer. No matter if it’s a syntax mistake, a logic flaw, or perhaps a deep architectural situation, every one can instruct you something useful when you go to the trouble to reflect and evaluate what went Mistaken.
Start out by inquiring you a couple of crucial issues as soon as the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with better practices like unit testing, code evaluations, or logging? The solutions usually reveal blind spots within your workflow or comprehension and allow you to Create more robust coding practices transferring ahead.
Documenting bugs will also be a wonderful pattern. Keep a developer journal or maintain a log in which you Observe down bugs you’ve encountered, the way you solved them, and Whatever you uncovered. With time, you’ll start to see patterns—recurring challenges or popular faults—you can proactively keep away from.
In crew environments, sharing Everything you've discovered from the bug with the friends might be Specially effective. Regardless of whether it’s through a Slack information, a short write-up, or A fast expertise-sharing session, assisting others steer clear of the identical issue boosts workforce effectiveness and cultivates a stronger Mastering tradition.
More importantly, viewing bugs as classes shifts your state of mind from frustration to curiosity. In place of dreading bugs, you’ll commence appreciating them as essential portions of your advancement journey. After all, several of the very best builders aren't those who create great code, but individuals who continuously understand from their mistakes.
In the long run, each bug you correct provides a fresh layer towards your skill established. So next time you squash a bug, take a minute to reflect—you’ll arrive absent a smarter, more capable developer thanks to it.
Conclusion
Strengthening your debugging competencies will take time, exercise, and patience — nevertheless the payoff is large. It makes you a more productive, self-assured, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be improved at what you do.